Drawing Sprites Along a Line2D in Godot
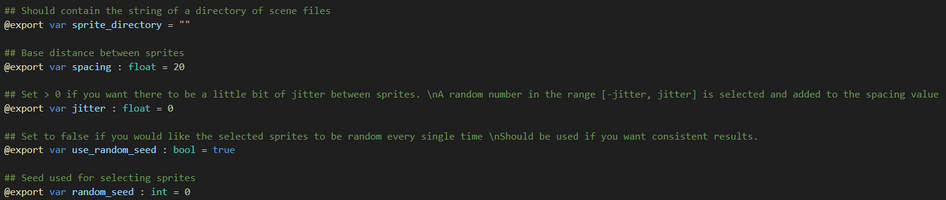
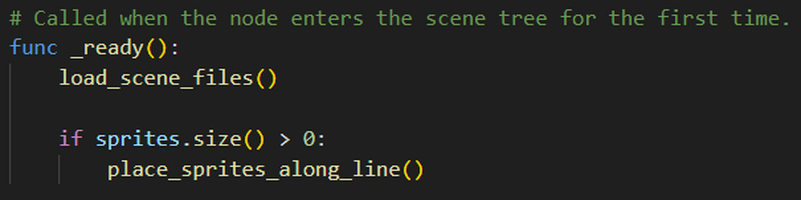
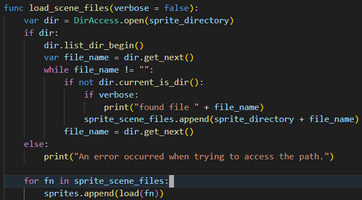
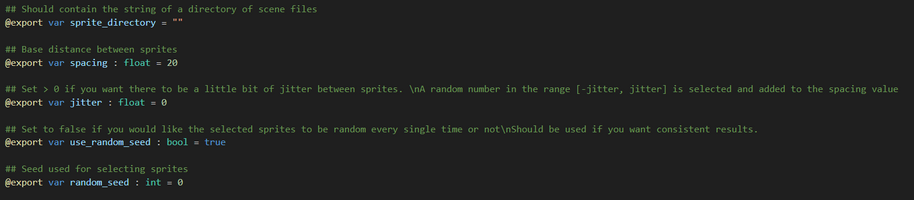
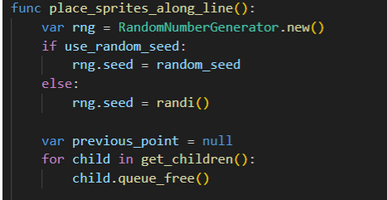
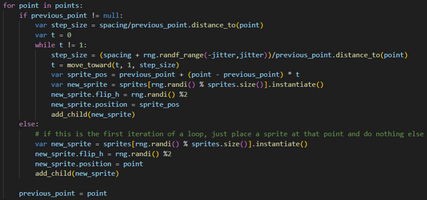
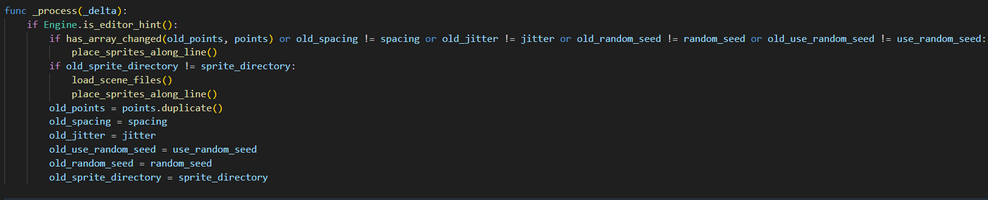
Here is an explanation of a Godot tool I made during Learn You a Game Jam 2024 that draws sprites along a Line2D.
If you'd like to use this in your own projects, I've uploaded it to my Github
The tool takes advantage of the Line2Ds intuitive editing of it's "points" variable and uses the values in that variable to place randomly selected sprites at a configurable number of steps along the line. In FEED, I use this effect to place grass sprites, but the concept could be applied to other decorative sprites as well.
I wanted to make this as a way of learning how to use the "@tool" feature of Godot, the YouTube videos I found for this feature made it seem way more confusing than it needed to be and I found the Godot documentation to be by far the most helpful resource. Simple and to the point.
A normal Godot script runs it's code while your game is running, by calling _process() (among other functions) every frame. A tool script is a script that runs not only during gameplay, but also in the editor. This lets you write code that helps you build out scenes and modify other nodes in your scene in a way I hadn't ever used in a game engine before. The basic example given in the documentation is a sprite that rotates every frame, but tool scripts don't just have to modify the node it's attached to it can access the full scene tree and create other nodes just like any other script.
I use this in my SpriteLineDrawing tool and now I'll go through each part of it step-by-step:
For future reference, here is all of the exported variables that the user can configure directly in the editor:
When the tool is first added to the scene the "_ready()" function is called. Here is what the _ready() function looks like in my script:
As you can see, the first thing we have to do is load all the scene files in a function called "load_scene_files()"
load_scene_files() opens the directory given by the user, saves all the filenames to an array and then loops over that array and loads each scene file and stores a reference to them in an array called sprites. There's some basic error checking. Most of this code is just example code from the Godot documentation on directory access. One important note is that the directory has to be of the format "res://<directory>/.../"
Now for the sprite placement:
First we configure our RandomNumberGenerator, if the user sets "use_random_seed" to true, the RNG is seeded so it looks the same every time the scene opens, if you want it randomized, all you have to do is set "use_random_seed" to false and the seed will be randomly generated as well. Next, we free any existing children of the node in case there were sprites already drawn on the line.
Now we start looping through the points on the Line2D. These points are stored in an array called "points". In this tool, sprites are added as children to the node at positions determined by taking steps along a line. Lines (geometric, not Line2D) are made up of two points. However, on the first iteration of our loop through all of the points belonging to our Line2D we have only one point to work with, so we just place a sprite directly at that point and store that point in a variable called "previous_point". On the second iteration and going forward, we have two points, so we can step along the line formed from previous_point->point.
First we calculate our step_size using the "spacing" variable configured by the user. The step_size is calculated such that when if spacing is 100, and there are 500 units between previous_point and point there will be 5 sprites placed, one every 100 units. At every step, the sprite's position is calculated via interpolation using the formula found here. A sprite is selected at random from our sprites array and instantiated, then it is randomly flipped horizontally (just for a little more variation) and it's position is set to the position calculated earlier. Finally, it is added as a child to the line.
Updating Sprites In The Editor (In Real-Time)
That's about it! However, with just this code the sprites will only be drawn when you hit the scene is actually running. To have the code run in the editor you have to add the line "@tool" as the first line of code in the script. With this, the code will run when the scene is opened in the editor.
To have the values update in real-time in the editor I added some extra code to check if any exported variables have changed since the last time frame:
All of these "old_" variables are declared globally underneath my exported variables.
Get FEED
FEED
Creepy-cute 2D action-platformer with unique movement mechanics!
Status | Released |
Authors | JacobJustice, arxzie |
Genre | Platformer, Action, Survival |
Tags | Atmospheric, challenging, Creepy, Cute, Pixel Art, Singleplayer, Survival Horror |
More posts
- The Art of FEEDJul 03, 2024
- Basic 2D Rain, Thunder and Lightning in GodotJul 02, 2024
- Resources I Used to Learn Godot!Jul 02, 2024
- Gameplay Design - The Hunger MechanicJul 02, 2024
- Gameplay Design - Character Movement and CombatJul 01, 2024
- FEED - Concept to Final Product (Start here!)Jul 01, 2024
Leave a comment
Log in with itch.io to leave a comment.